Writing a function to add to a List
In this article we are going to show how to write a function that can add items to a list. Each time we run the function, a new item is added to a predefined list. The secret is to use “<<-” instead of “<-“, inside the function when adding items to the list. The same idea can be used to sequentially add items to other objects like vectors and matrices, data frames, etc…
The following command defines an empty list by the name “A”. Later on we will start adding items to this list.
A<-list()
The next code, defines a function by the name of “Add.Item”. This function will add a vector made up of 3 random numbers at the end of the list “A”. Note that “A[[length(A)+1]]” represents the next item of “A” and “runif(3,0,1)” represents a vector of 3 random numbers between 0 and 1. Hence “A[[length(A)+1]]<<-runif(3,0,1)” is the command that will append a vector of 3 random numbers to the list “A”. In general, you can change “runif(3,0,1)” with whatever you would like to add to the list.
Add.Item<-function(){A[[length(A)+1]]<<-runif(3,0,1)}
In order to add the first item to the list “A”, you need to run the function once, as follows.
Add.Item()
So now when we display the list “A”, we find that it has its first item.
A

Now we can run the function again in order to add another item (in our case a vector of 3 random numbers) to the list.
Add.Item()
A
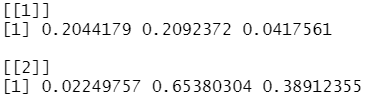
The last two lines of code can be repeated over and over again in order to populate the list with as much items as needed. One can further incorporate the function within a loop, in order to repeated the function automatically for many times.
for(i in 1:3){Add.Item()}
A
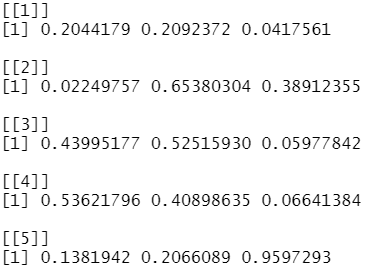